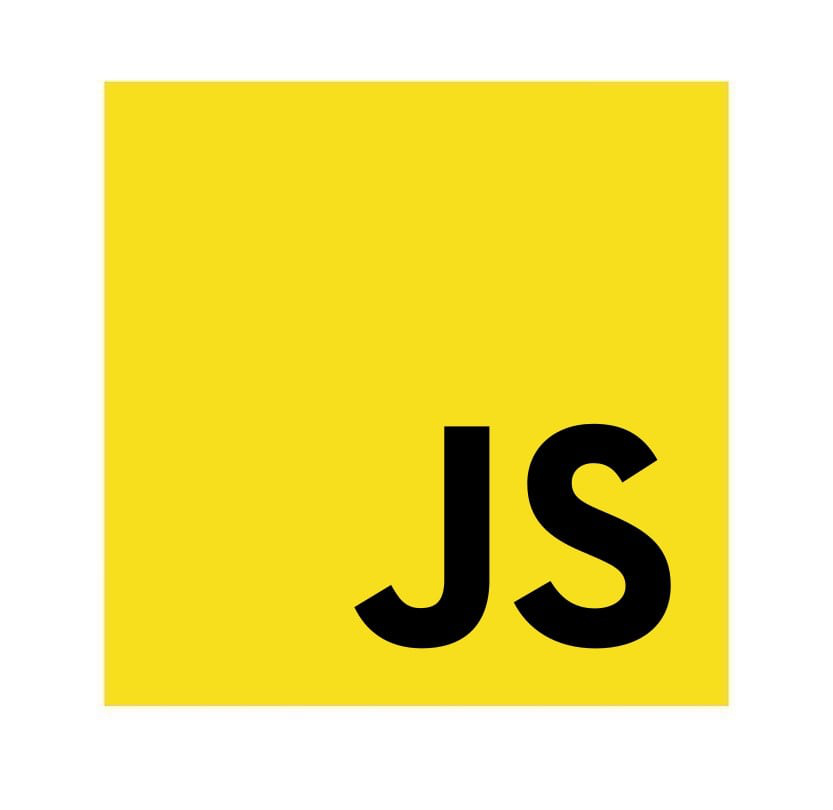
JavaScript
# ES6 Arrow Functions in JavaScript
Arrow Functions in ES6 have introduced a new way we can define our functions in JavaScript. This is highly popular as it provides us certain benifits and a shorter syntax to write our function definitions.
So normally we write a function like:
function answerToEverything() {
return 42;
}
answerToEverything(); // 42
Now let's try to convert the same into a arrow function:
var answerToEverything = () => 42;
answerToEverything(); // 42
We can already see that arrow functions provide us with a shorter and cleaner syntax of writing our functions. Now let's understand what we actually did to write our function as a arrow function.
We removed the keyword function
and introduced a (arrow) =>
after the input parameters parenthesis ()
. After the parenthesis we write the statements for our function and if its only a single statement as in the exmple above we can optionally remove the block parenthesis {
}
for more cleaner syntax.
(parameters) => { statements }
Let's see a few examples to make it clear how we can use arrow functions with different parameters and statements:
- No parameters
- Single parameter
- Multiple parameters
- Single statement
- Multiple Statements
# No parameters
If there are no parameters we can use empty parentheses ()
befoe the arrow.
() => 42;
We can also write them without parenthesis using a _
as:
_ => 42;
# Single parameter
When we have a single parameter the parenthesis are optional and we can write it both with or without the parenthesis.
(a) => a + 42;
a => a + 42;
Both will work in the same way where whatever value of a
we pass it will add 42 to it and return the value.
# Multiple parameters
For multiple parameters we will pass the parameters seperated by comma ,
inside our parentheses ()
.
(a, b) => a + b;
# Single statement
For single statement we don't need to create a block with {
& }
which is optional.
() => 42;
but using block with {
& }
is also valid and then we would have to use return
as well.
() => {
return 42;
};
# Multiple Statements
For multiple statments we have to use a block using {
& }
.
(a, b) => {
var c = a + b;
c + 42;
return c;
};
# Arrow functions are Syntactically Anonymous
Now from the above examples we can observe that we don't have any name for our arrow funtions which we can use to call them i.e they are anonymous. So to access our arrow funtions we generally assign them to a variable and use them.
var answerToEverything = () => 42; // assigning to a variable
answerToEverything(); // 42
# Arrow functions don't have self-referencing ( No binding of this
)
In Arrow Functions this
is lexically bound i.e. it uses this
from the code that contains the arrow function. Therefore Arrow functions don't have its own binding of this like regular function expressions which have their on this
so we have to explicitly pass the this
context to function of the containing block if we require it inside the function.
This is really helpful when working with promises and callbacks to handle data and response.
// ES5
ExampleAPI.get = function(endpoint) {
var that = this;
return new Promise(function(resolve, reject) {
http.get(that.base_url + endpoint, function(data) {
resolve(data);
});
});
};
// ES6
ExampleAPI.get = function (endpoint) {
return new Promise((resolve, reject) => {
http.get(this.base_url + endpoint, function(data) {
resolve(data);
});
});
};
Here, we are able to access the base_url
directly and we don't have to explicitly pass the this
context.
Learn More
- ES6 Arrow Functions in JavaScript