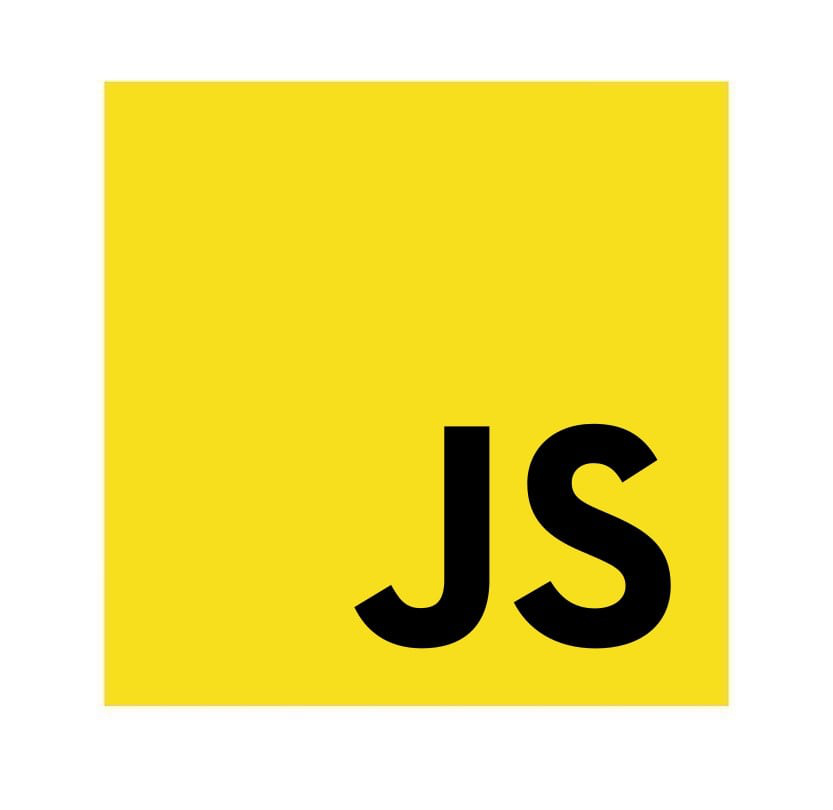
JavaScript
# Events in JavaScript - Crash Course
One of the most important concept in JavaScript and web development is events and how they are used to create a whole user experience for a product or a website based on user interaction and website flow.
In this crash course we will cover the following topics to understand and manipulate events in JavaScript:
- What are events in JavaScript?
- What are event handlers? How to use event handlers?
- What are event objects?
- What is event bubbling & event capture?
- Event Bubbling
- Event Capture
- Prevent Default Behavior with
preventDefault()
- Event Delegation
- Stopping an Event Propagation using
stopPropagation()
# What are events in JavaScript?
Events in simple terms are things that happens or takes place at specific times or with specific actions.
In JavaScript we interact with HTML through events that occur when the user or the browser manipulates a web page. When the page loads it is an event and when a user clicks a button on the page, that is also an event.
Based on type and functionalities we have different types of events available to us, listing below some of the most used events in JavaScript Programming:
1. Mouse Events
These events are related to mouse activity of the user on a web page, like clicking, hovering, moving the mouse pointer etc.
Event | Description |
---|---|
click | When the mouse clicks on an element. |
contextmenu | When the mouse right-clicks on an element. |
mouseover | When the mouse cursor comes over an element. |
mouseout | When the mouse cursor leaves an element. |
mousedown | When the mouse button is pressed over an element. |
mouseup | When the mouse button is released over an element. |
mousemove | When the mouse is moved. |
2. Keyboard Events
These events are related to keyboard activity of the user on a web page, like pressing a key, releasing a key etc.
Event | Description |
---|---|
keydown | When any key is pressed on the keyboard |
keypress | When any key (except Shift, Fn, or CapsLock) is in pressed position. |
keyup | When any key is released on the keyboard |
3. Document Events
These events are associated with the working of a webpage in a browser.
Event | Description |
---|---|
DOMContentLoaded | when the HTML is loaded and processed, DOM is fully built. |
4. From Events
These events are related to HTML form submissions or while working with input fields.
Event | Description |
---|---|
submit | When the HTML form is submitted |
focus | When any element is in focus |
5. CSS Events
These events are related to various CSS events that might occur while working with CSS animations or transitions.
Event | Description |
---|---|
animationstart | A CSS animation has started. |
animationend | A CSS animation has completed. |
transitionstart | A CSS transition has started. |
transitionend | A CSS transition has completed. |
Apart from these 5 categories various other types of events are also available in JavaScript. Click here for a complete list of events that are available in JavaScript.
# What are event handlers? How to use event handlers?
Now that we know that we have different types of events available to us in JavaScript to create interactions and perform actions using events across our web application.
The next question is how to use these events to create custom actions and interactions for our web application. To do this we have event handlers & event listeners available to us, so that we can write logic and trigger various actions on events.
# 1. Using Event Handler Properties
There are a number of ways in which you can add event listener code to web pages so that it will be run when the associated event fires.
Inline Attributes
A handler can be set in HTML with an attribute named on<event>
, where <event>
is the event you want to handle from the list of events available above.
Example: We want to execute a function helloWorld()
when we click on the button with text Hello!
defined in our HTML. We will use the click
event by defining a attribute onclick
on the HTML element and assigning the function we want to execute to it.
<button onclick="helloWorld()">Hello!</button>
function helloWorld() {
console.log('Hello World!');
}
Element Properties
This way is similar to the one we discussed above with the only difference being we avoid using HTML attributes directly and use pure JavaScript to add event handlers to our HTML elements.
Example: Taking the same example, instead of using the HTML attributes we will get the element we want to attach event to using DOM Access methods like getElementById()
, getElementsByClassName()
, getElementsByTagName()
, querySelector()
, querySelectorAll()
. Click here to read more about these methods.
Let's use querySelector()
to get access to the button element that we have in our HTML. Then we can access its properties and attach the function helloWorld()
on the event property onclick
.
<button>Hello!</button>
const btn = document.querySelector('button');
function helloWorld() {
console.log('Hello World!');
}
btn.onclick = helloWorld;
Note: This method is preferred over using inline attributes as it is more encapsulated keeping JavaScript and HTML separate which increases maintainability of larger codebase and provides more flexibility to alter and update events.
# 2. Using addEventListener()
and removeEventListener()
JavaScript also provides us with methods to add and remove events using event listeners methods
addEventListener()
- Attach an event handler to the specified element.removeEventListener()
- Remove an event handler that has been attached.
Learn More
- Events in JavaScript - Crash Course