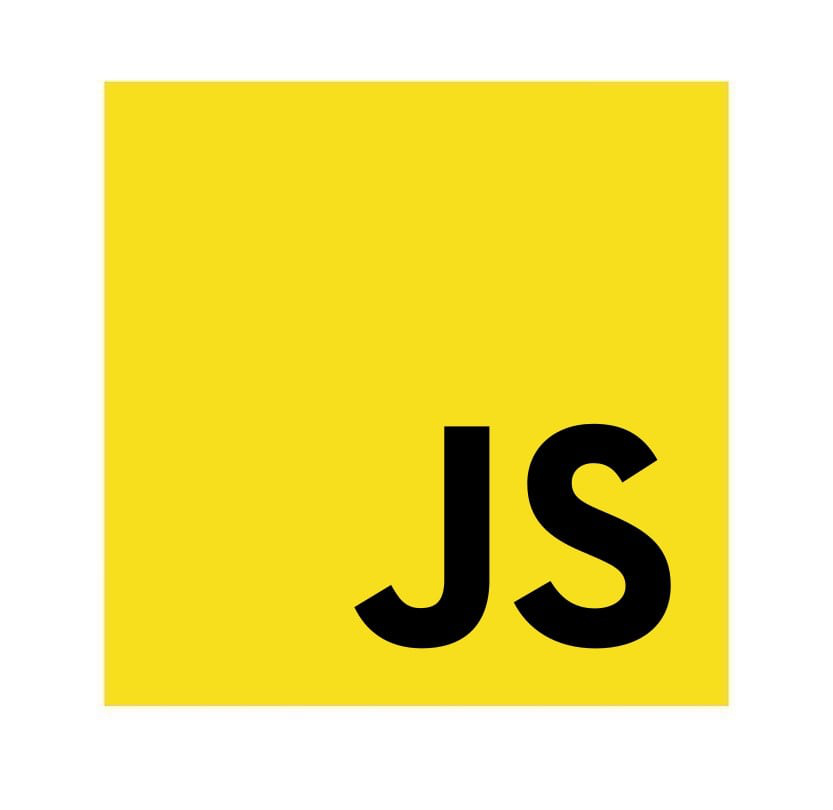
JavaScript
# JavaScript Interview Output based Questions and Answers 101
Let's look at some of the essential JavaScript questions which involve concepts which are frequently asked in interviews.
# What will be the output of the following statements and why?
console.log(1 < 2 < 2);
The output will be
true
because JavaScript will start from the left and start comparing the first two values which is1 < 2
where 1 is less than 2, so the output of the expression will betrue
.Now after evaluating the first expression we have the
true < 2
as the final expression to evaluate. As we are comparing a boolean and a number value by using the concept of Type Conversion, JavaScript will try to convert the boolean value to a number so that the comparison can be made. Astrue
translates to1
i.e. we now have1 < 2
as the final expression. So the final result evaluates totrue
.let a = 10; let b = 20; console.log('result is ' + a + b);
The output will be
'result is 1020'
. This also beacuse of the concept of Type Conversion. JavaScript will start from the left and the first thing it encounters a string'result is'
, next it has a expression to solve where it needs to add a string to the number 10. So it will convert the number 10 to a String and concatinate it with'result is'
, so the result will beresult is 10
and the exprssion now is'result is 10' + b
. Now same thing will happen again and the final output will be'result is 1020'
console.log(square(5)); function square(n) { return n * n; }
Here we have a console statement where we are calling the function
square(5)
by passing the value 5, After that we have the function definition ofsquare
. So the first thought that comes to mind is that this will give an error as we are calling a function before its definition is defined. But what will happen is we will get the output as25
i.e. the function will get executed without any problem.Why this happened? This is because of a concept in JavaScript called
Hoisting
which can be divied into two parts:1. Funtion Hoisting: The definition of all the functions are hoisted (lifted to the top of their functional/local scope), therefore they are accessable even if we call a function before we write its definition in the program.
2. Variable Hoisting: All varibables defined with
var
keyword are also hoisted (lifted to the top of their functional/local scope) but with a value ofundefined
.console.log(square(5)); var square = function(n) { return n * n; }
Now as we have seen in the previous question, here the concept of Variable Hoisting will take place and
square
will have a value of undefined untill the initialisation happens and a function definition is assigned to it. Therefore the console statement will result in a error thatUncaught TypeError: square is not a function
as it has the valueundefined
.const arr = [10, 12, 15, 21]; for (var i = 0; i < arr.length; i++) { setTimeout(function() { console.log('The index of this number is: ' + i); }, 3000); }
The output will be 4 times
The index of this number is: 4
after 3 seconds. This is because the for loop executes 4 times for the following values i: 0, 1, 2, 3 and when value of i is 4 the loop terminates. Now after the loop and exited there are 4setTimeout
calls in the execution stack of JavaScript which will execute after 3 seconds. Therefore after 3 seconds all 4 calls are executed one by one and we see the output 4 timesThe index of this number is: 4
as the last value of i is 4.Now if we want to log the output as:
The index of this number is: 0
The index of this number is: 1
The index of this number is: 2
The index of this number is: 3
We can achiveve this by changingvar
tolet
, becacuselet
has a blocked scope and it retains its value inside the execution context of the for loop.var array1 = ['a','b','c','d','e','f']; var array2 = array1; array2.length = 0; console.log(array1, array2);
The output will be
[], []
because we have declared a arrayarray1
which has the elements['a','b','c','d','e','f']
, next we assigned it toarray2
. Now as array is not a primitve data type it will create a reference and botharray1
andarray2
will point to the same set of array elements in the memory. When we make length ofarray2
as 0, we are making the array empty, so botharray1
&array2
will be empty arrays.let output = (function(x){ delete x; return x; })(3); console.log(output);
The output will be
3
because firstly we have madeoutput
varibale equal to a IIFE - Immediately Invoked Function Expression which will execute with the value ofx
as3
. Now thedelete
operator only works with objects and is used to delete keys from a object. Therefore the function will return 3 and the value 3 will be assinged tooutput
variable.
Learn More
- JavaScript Interview Output based Questions and Answers 101