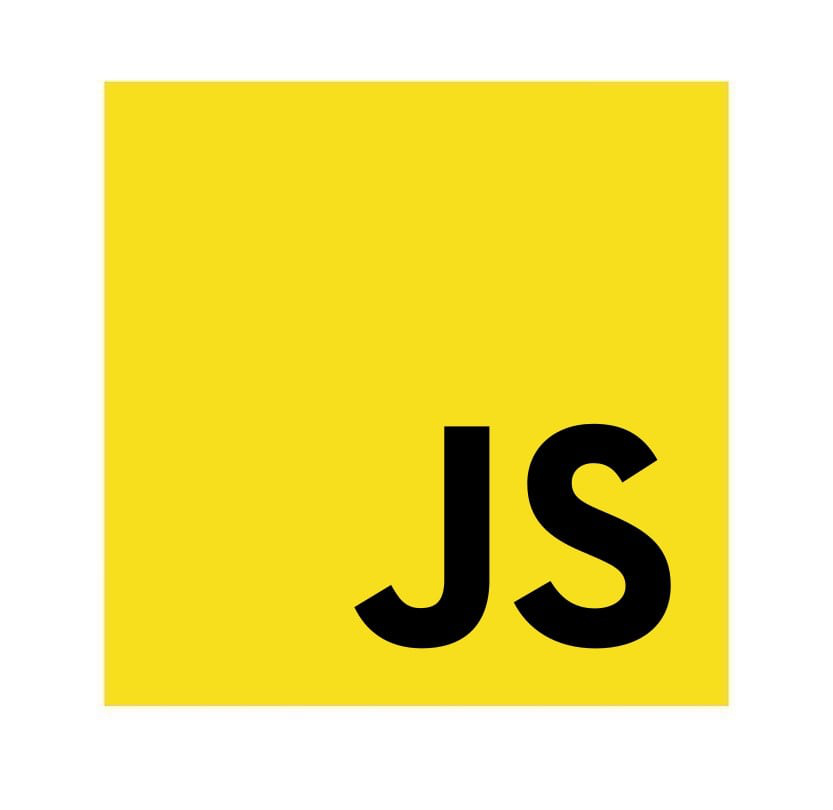
JavaScript
# Understanding Spread Operator in JavaScript ES6
ES6 introduced the spread operator in JavaScript which is represented by ...
(three dots) and helps an expression to be expanded in places where multiple elements or variables or arguments are expected.
Let's take an example and see what happens when we use the spread operator:
const array1 = [1, 2, 3];
const array2 = [...array1, 4, 5];
console.log(array2); // Output: [1, 2, 3, 4, 5]
In the above example we see that while creating an array we can add multiple elements to the array and if we want to add elements of array1
to the starting of array2
we can use the spread operator to achieve this which will expand the elements of array1
inside array2
.
This is one way we can use the spread operator. Let's look at different ways in which spread operator is useful:
Arrays
- Combine arrays
- Copy array
- Concatenate arrays
- String to array
Objects
- Combine objects
- Copy objects
- Concatenate objects
Function Arguments
- Replace
apply()
- Apply for
new
- Replace
# Arrays
We have already seen an example of how we can use spread operator while working with arrays, let's cover all cases with examples.
# Combine Arrays
We can combine arrays using the spread operator as we have already seen.
const array1 = [1, 2, 3];
const array2 = [...array1, 4, 5];
console.log(array2); // Output: [1, 2, 3, 4, 5]
# Copy Array
We can create unique copy of a array using the spread operator where the copied array and the original array will have different references in the memory.
const array1 = [1, 2, 3];
const copyarray1 = [...array1];
console.log(copyarray1); // Output: [1, 2, 3]
copyarray1.push(4);
console.log(array1); // Output: [1, 2, 3]
console.log(copyarray1); // Output: [1, 2, 3, 4]
# Concatenate Arrays
We can replicate the functionality of Array.prototype.concat()
which is used to add elements of one array to the end of another array. Below is an example using Array.prototype.concat()
:
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
array1 = array1.concat(array2);
console.log(array1); // Output: [1, 2, 3, 4, 5, 6]
Now let's replicate the same using spread operator.
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
array1 = [...array1, ...array2];
console.log(array1); // Output: [1, 2, 3, 4, 5, 6]
# String to Array
One unique use case is we can use the spread operator to convert characters of a string into individual array elements.
let str = "codewhoop";
let characters = [...str];
console.log(characters); // Output: ['c', 'o',' d',' e', 'w', 'h', 'o', 'o', 'p']
# Objects
We can also use spread operator with objects in a similar way as we did with arrays. Let's understand by taking examples.
# Combine Objects
We can combine objects using spread operator as we did while working with arrays.
let object1 = {a: 1, b: 2};
let object2 = {...object1, c: 3};
console.log(object2); // Output: {a: 1, b: 2, c: 3}
# Copy Objects
We can make a shallow-copy (excluding prototype) using spread operator which is a shorter syntax than Object.assign()
.
let object1 = {a: 1, b: 2};
let object2 = {...object1};
console.log(object2); // Output: {a: 1, b: 2}
object2.c = 3;
console.log(object1); // Output: {a: 1, b: 2}
console.log(object2); // Output: {a: 1, b: 2, c: 3}
# Concatenate Objects
We can also Concatenate two or more objects using the spread operator.
let object1 = {a: 1, b: 2};
let object2 = {c: 3, d: 4};
object1 = {...object1, ...object2};
console.log(object1); // Output: {a: 1, b: 2, c: 3, d: 4}
# Function Arguments
We can also use the spread operator to pass multiple arguments to a function.
# Replace apply()
Earlier we had Function.prototype.apply()
if we wanted to use element of an array as arguments of a function call. We can achieve the same functionality using the spread operator. Let's take a example to see both the approaches:
function example(a, b, c) {
return a + b + c;
}
let args = [0, 1, 2];
example.apply(null, args); // Returns: 3
example(...args); // Returns: 3
this can be very useful when we are working with inbuilt functions like Math.max()
, Math.min()
, Math.hypot()
etc. which take multiple arguments.
let array1 = [2, 4, 8, 6, 0];
let max = Math.max(...array1);
console.log(max); // Output: 8
# Apply for new
When we have to call a constructor with new
it's not possible to directly use an array as arguments with Function.prototype.apply()
as apply()
does a call and not a construct. This problem is solved with the spread operator as we can use it with both a call and a construct.
let dateData = [2019, 9, 29];
let d = new Date(...dateData);
console.log(d); // Output: Tue Oct 29 2019 00:00:00 GMT+0530 (IST)
So that was all for the spread operator.
Learn More
- Understanding Spread Operator in JavaScript ES6