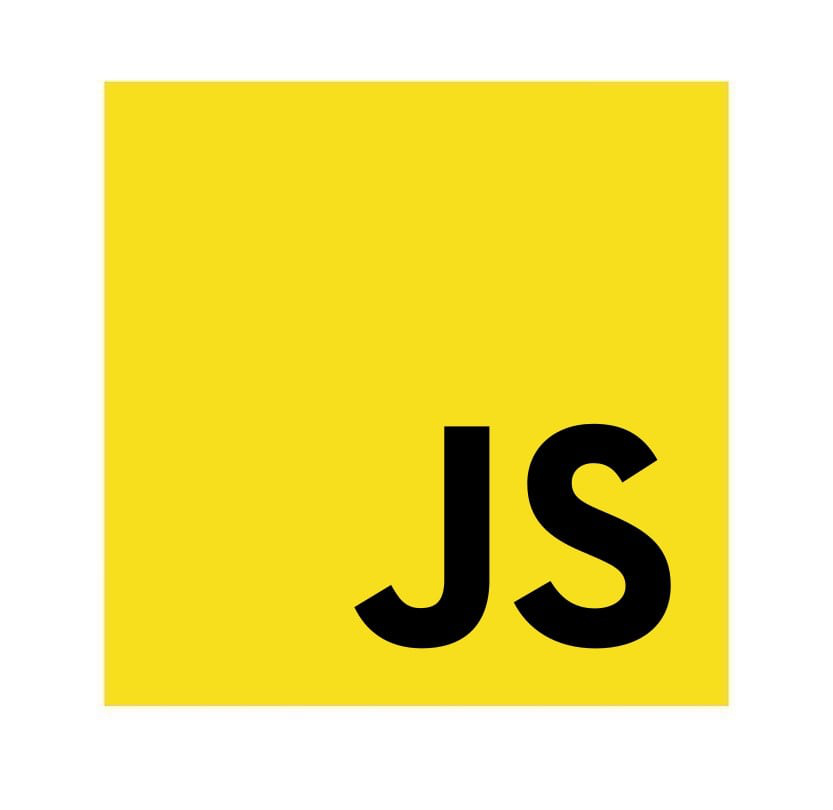
JavaScript
# var vs let vs const
Two new ways to create variables were introduced in ES2015 (ES6) which are let
and const
, So now we have three ways for creating variables:
var
let
const
Before comparing them, let's understand some concepts associated with variables in JavaScript.
- Declaration and Initialization
- Scope of a variable
- Hoisting
# Declaration and Initialization
Declaration of variable is when we create a variable in JavaScript it creates a new identifier with the variable name and assigns it a value of undefined
. e.g.
var a;
console.log(a); // undefined
Here we have declared a variable a
but we have not assigned any value to it. So the identifier a
will be created with value undefined
and in the second line when we log that varibable we will get undefined
.
Initialization of a variable is when we first assign a value to the variable. Re-initialization is when we update the previously assigned value.
var a; // Declaration
a = 1; // Initialization
a = 2; // Re-initialization
# Scope of a variable
Scope of a variable is where in the program the variable is accessible. In JavaScript we have three types of scope:
- Global Scope - Any variable which is not inside any function or block (a pair of curly braces) is said to be in the global scope. The variables in global scope can be accessed from anywhere in the program.
- Function Scope - Any variable which is declared inside a function is in the function scope, i.e. they can only be accessed within that function.
- Block Scope - A blocked scoped variable is accessible only inside the nearest pair of curly brackets (
{
&}
).
# Hoisting
As we discussed earlier that in JavaScript when we declare a variable it is assigned a value of undefined
before it is initialized with a value.
# var vs let vs const
Now let's list down the differences between the three ways of creating variables and then we will explain each one in detail:
var | let | const | |
---|---|---|---|
Scope | Function Scope | Block Scope | Block Scope |
Hoisting | Yes | No | No |
Re-initialize | Yes | Yes | No |
# Scope - var, let and const
Now, var
has function scope which means that if we declare a variable inside a function we cannot access it out side of the function, also let
and const
have blocked scope which means they are accessible only inside the nearest pair of curly brackets ({
& }
). So let's take an example to understand better.
function scope_example(){
var a = 1;
let b = 2;
const c = 3;
console.log(a); // 1
console.log(b); // 2
console.log(c); // 3
}
scope_example();
console.log(a); // ReferenceError: a is not defined
console.log(b); // ReferenceError: b is not defined
console.log(c); // ReferenceError: c is not defined
Now, when we execute this we see that inside the function we get the values of all the three variables and outside we get an error ReferenceError
that all the variables are not defined.
Now let's check for the blocked scope for all three variables:
if(true){
var a = 1;
let b = 2;
const c = 3;
console.log(a); // 1
console.log(b); // 2
console.log(c); // 3
}
console.log(a); // 1
console.log(b); // ReferenceError: b is not defined
console.log(c); // ReferenceError: c is not defined
Comparing from before we see that var
variable is accessible outside the if block but let
and const
variables give ReferenceError
which shows that let
and const
have block scope where as var
has function scope.
# Hoisting - var, let and const
Only var
is hoisted which means that it is assigned a value of undefined
untill it is initialized with a value. Let's take a example to confirm this.
console.log(a); // undefined
console.log(b); // ReferenceError: Cannot access 'b' before initialization
console.log(c); // ReferenceError: Cannot access 'c' before initialization
var a = 1;
let b = 2;
const c = 3;
We see that the value of a
is logged as undefined
and we get a reference error in case of let
and const
that they cannot be accessed before initialization.
# Re-initialize - var, let and const
Re-initialization is when we update the previously assigned value of a variable.
var a = 1;
let b = 2;
const c = 3;
a = 10;
b = 20;
c = 30; // TypeError: Assignment to constant variable.
We see that we are able to re-initialize a
and b
but when we try to re-initialize c
we get a type error. Therefore we cannot re-initialize a const
variable after it is initialized.
This sums up the basic difference between var
, let
and const
and considering this we can make better judgement of when to use which type.
Learn More
- var vs let vs const