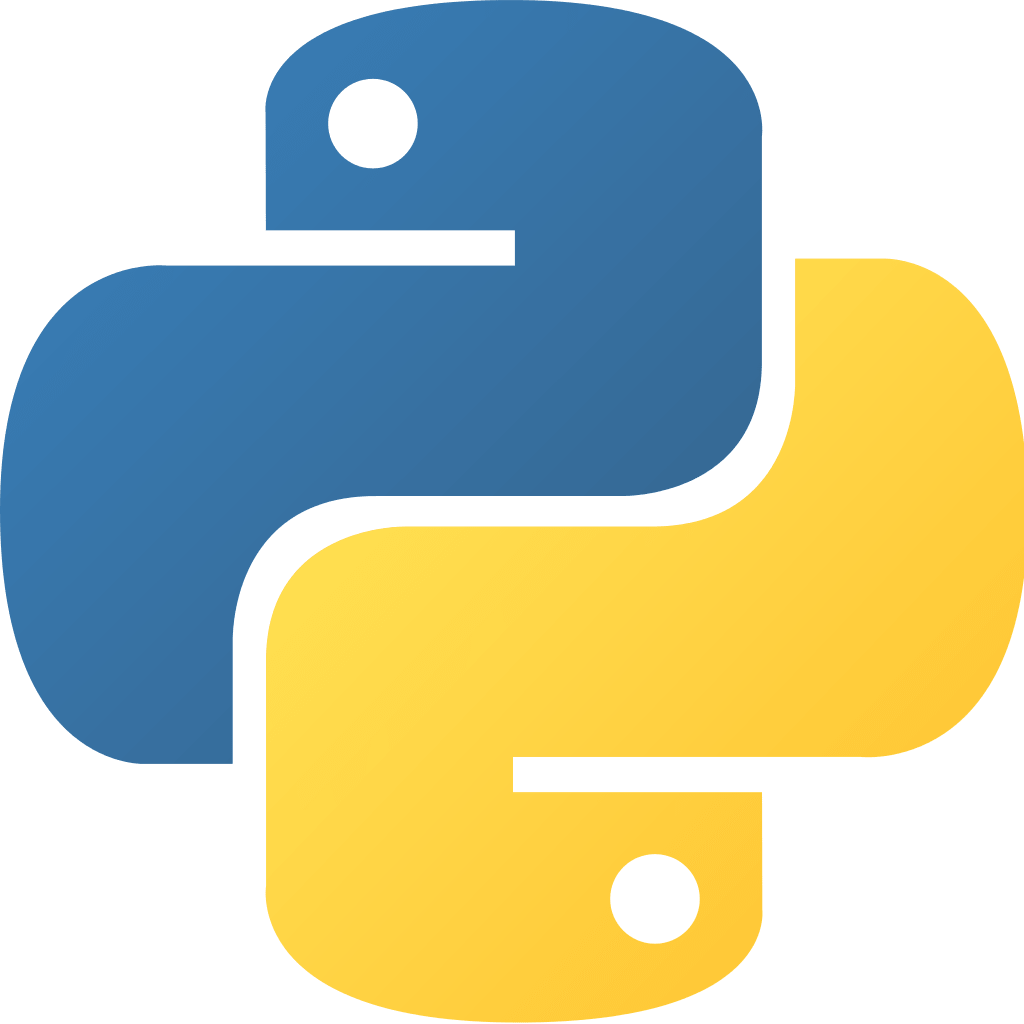
Python
# Integer input using raw_input
Taking Integer input using raw_input()
in Python.
Python language has a lot of cool inbuilt features which makescoding in python time effcient and also the code is almost reducedto half of the length it would have been in C++/C (though I dont have anything against them 😛 ).
This article is about taking integer input in Python which would cover 3 things:
- Taking single integer input
- Taking multiple integer inputs in one line
- Taking integer array as input in one line
Let us start by taking a single integer input,
input = int(raw_input())
For example,we entered 6 from the keyboard,
raw_input()
takes input from the keyboard in string format i.e "6" and int(raw_input())
then typecasts the input from string to an integer i.e. 6
Moving on to taking delimiter(',',' ') separated multiple inputs in a single line,
input1,input2 = map(int,raw_input.split())
For example, we entered 6 5 from the keyboard:
raw_input()
takes input from the keyboard in string format i.e "6 5"raw_input().split()
splits the string by space " " and change it into "6" "5"map(int,raw_input().split())
typecasts all the inputs to integers i.e6
5
and store ininput1
andinput2
Lastly,taking delimiter (',',' ') separated array as input in single line.
It is same as multiple inputs with the difference that on the left of "=" is our array.
inputArray = map(int,raw_input.split())
This automatically takes input ,splits it,typecasts in integer ands stores as an array It means that using this single line,you can eliminate the use of any loop for taking an array input.
You can also enter "," (comma) separated input and just provide the input in split as
inputArray = map(int,raw_input.split(','))
which is by default " "(space character)
Hope this helps 😃