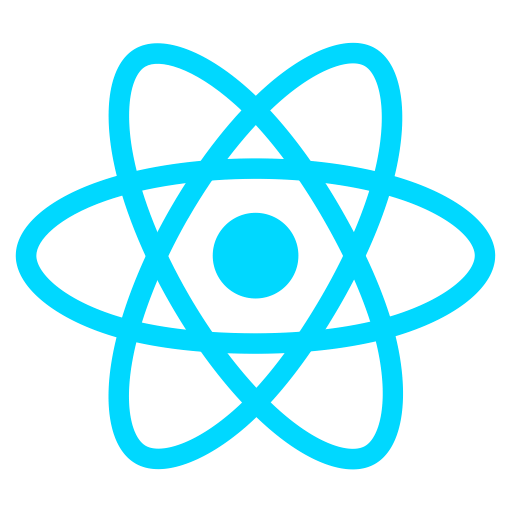
React JS
# Understanding React Component Lifecycle
Components in React have a lifecycle which we can manage and manipulate for different purposes. React component’s lifecyle methods can be split in four stages:
- Initialization - set default state and props.
- Mounting - component mounts on the DOM (First render).
- Updating - if state value is changed and component needs to re-render or update.
- Unmounting - component unmounted from the DOM.
Note: We can combine Initialization and Mounting in one stage as well, which is represented at most places, but for understanding better we will take out the Initialization stage from Mounting.
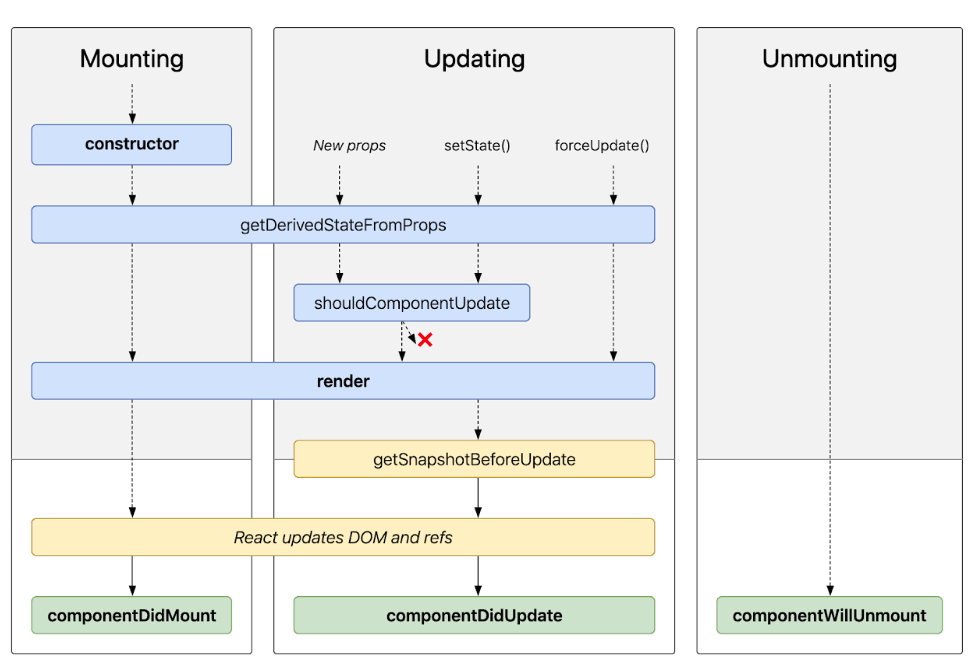
# Initialization
The initialization stage is where the default values of the state
and props
are set for component. There are two ways to initialize state in a React component:
- Using constructor
- Directly inside the class
# Using constructor
Using constructor we can set initial state beacuse when the component class is created, the constructor is the first method which is called.
class App extends React.Component {
constructor(props) {
// calling the parent class constructor
super(props);
// setting default state
this.state = {
flag: false,
name: "Codewhoop"
}
}
render() {
}
}
Note: When you write your own constructor it is important to call the parent class constructor using super()
as the default implementation calls it automatically and if you are overwriting the default constructor its important to call super()
to prevent bugs if the parent was required to do some initialization before the child component.
# Directly inside the class
We can directly define default values inside the class by setting the state
object.
class App extends React.Component {
// setting default state
state = {
flag: false,
name: "Codewhoop"
}
render() {
return (
<p>Codewhoop</p>
)
}
}
We are not using constructor here and its a much cleaner way of defining default state
values. In this method the state
property is referenced directly i.e without this
and we can still access this.props
inside the state object.
# Mounting
Mounting takes place after the initialization and at this stage the react component mounts on the DOM i.e. it is rendered for the first time.
The methods that we have during this stage in sequence of when they are executed are:
static getDerivedStateFromProps()
render()
componentDidMount()
static getDerivedStateFromProps()
- This method is called right before calling the render method, both on the initial mount and on subsequent updates. It returns an object to update the state, or null if there is nothing to update.
static getDerivedStateFromProps(props, state) {
// Custom behaviour
return null;
}
render()
- This method returns the JSX which is rendered on to the DOM.
render() {
return (
// JSX
)
}
componentDidMount()
- This method is called immediately after a component is mounted on the DOM. It is most commonly used to get data from a endpoint.
componentDidMount(){
// Custom behaviour
}
Earlier we had a method
componentWillMount()
which is now declared unsafe and can be used asUNSAFE_componentWillMount()
but we should avoid using it in our code.
# Updating
This stage is when the component needs to update or re-render which is when data of the component (state or props) updates in response to user events like clicking, typing etc. The methods that are available in this stage in the order of execution are:
static getDerivedStateFromProps()
shouldComponentUpdate()
render()
getSnapshotBeforeUpdate()
static getDerivedStateFromProps()
- This method is called right before calling the render method, both on the initial mount and on subsequent updates. It returns an object to update the state, or null if there is nothing to update.
static getDerivedStateFromProps(props, state) {
// Custom behaviour
return null;
}
shouldComponentUpdate()
- The default behaviour of this method is to re-render on every state change and we can customise the behaviour if we want to not update in some cases by returningfalse
. This method is not called in initialization stage and is called before render in updating stage.
shouldComponentUpdate(nextProps, nextState){
// Custom behaviour to return true or false
return true;
}
render()
- This method returns the JSX which is rendered on to the DOM.
render() {
return (
// JSX
)
}
getSnapshotBeforeUpdate()
- This method is invoked right before the current rendered output is reflected on the DOM. It enables your component to capture some information from the DOM (e.g. scroll position) before it is potentially changed. Any value returned by this method can be passed as a parameter tocomponentDidUpdate()
assnapshot
.
getSnapshotBeforeUpdate(prevProps, prevState) {
// Custom behaviour
return null;
}
componentDidUpdate()
- This method is invoked right after updating is complete. This method is not called for the initial render. We can use this method to operate on the DOM when the component has been updated. One can also usesetState()
in this method but it must be wrapped in a condition to avoid a loop of component updation.
componentDidUpdate(prevProps, prevState, snapshot) {
// Custom behaviour
}
# Unmounting
It is the stage where the component is unmounted from the DOM. Only one method is available during this stage which is componentWillUnmount()
.
componentWillUnmount()
- It is called immediately before the component is unmounted from the DOM and is used to perform any cleanup operations, such as clearing timers or removing any DOM element that were created.
componentWillUnmount(){
// Custom behaviour
}
So, this sums up the overall lifecycle picture of a component divided into four stages.
Learn More
- Understanding React Component Lifecycle