# Linked List Basics
Linked list is a linear data structure which is made up of nodes connected together by pointers.
Each node has two main parts :
- Data - contains the data/value to be stored
- Link – contains address of the next node.
Each Node in a Linked List is created Dynamically.
A head or start pointer is used to keep track of the starting of linked list.
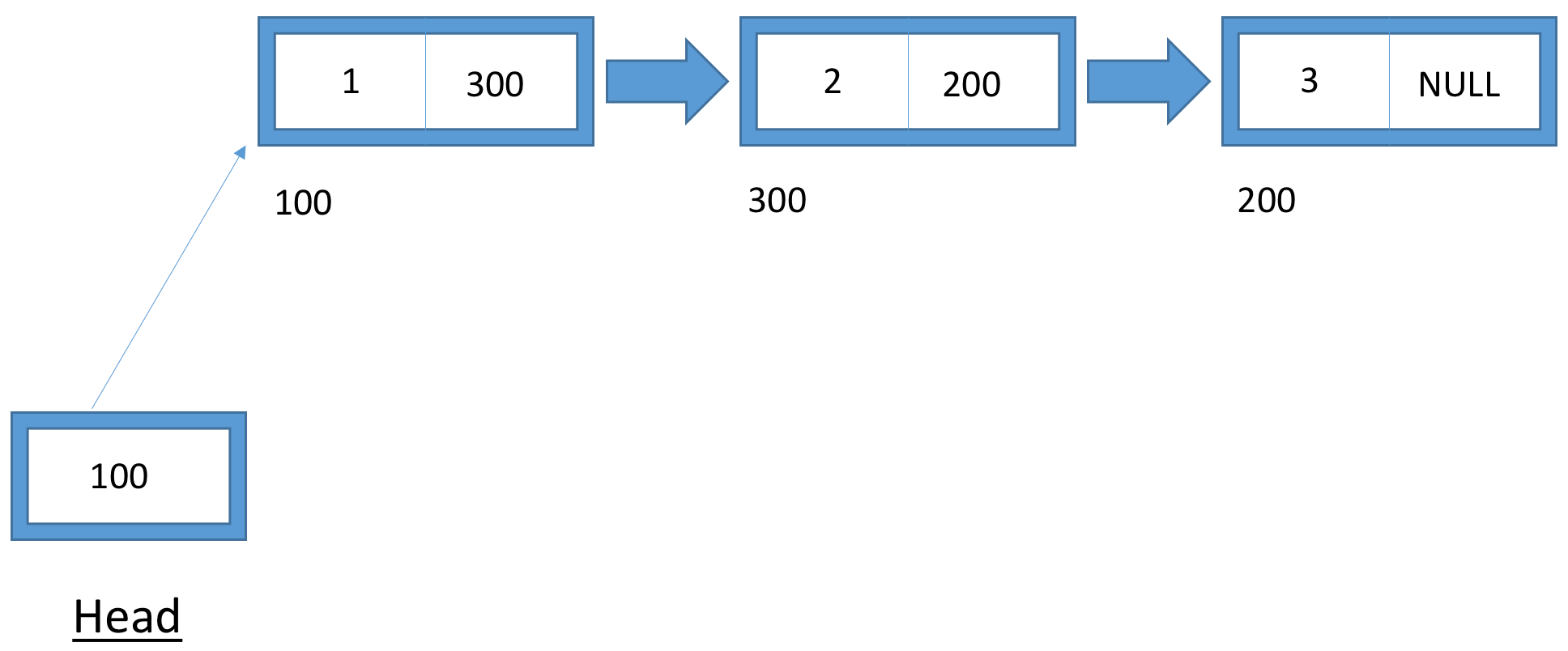
# Source Code - C++
#include <iostream>
using namespace std;
//Creating Node Structure
struct Node{
int data;
Node *link;
};
//creating head pointer and equating to NULL
Node *head=NULL;
//Main Method
int main()
{
//Creating a new Node
Node *ptr=new Node();
//Adding data and setting link to NULL
ptr->data=2;
ptr->link=NULL;
//Pointing head to created Node
head=ptr;
cout<<"data is"<<head->data;
return 0;
}