# Linear Search
Linear Search is a searching algorithm in which we sequentially check each and every element until we find the element we are looking for or we reach the last element and the element is not present.
Suppose we are looking for element 4 in the given array.
Element 4 found at index 3.
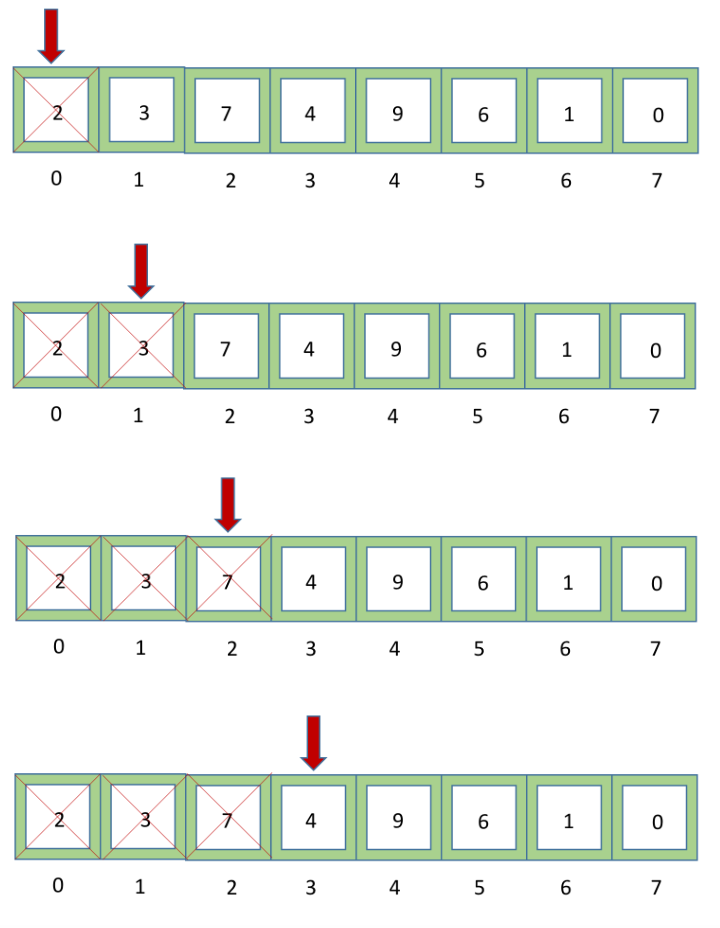
# Source Code - C++
#include <iostream>
using namespace std;
//Function to perform Linear Search
int LinearSearch (int A[], int n, int e)
{
int i, found= -1;
for(i=0 ; i<n; i++)
{
if( A[i] == e )
{
found=i;
break;
}
}
return found;
}
//Main Function
int main() {
int n;
cout<<"enter size of array\n";
cin>>n;
int A[n],e,i,ans;
cout<<"enter elements of array\n";
for(i=0;i<n;i++)
cin>>A[i];
cout<<"enter element to be found\n";
cin>>e;
ans=LinearSearch(A,n,e);
if(ans==-1)
cout<<"not found\n";
else
cout<<"Found at index:"<<ans;
return 0;
}
Time Complexity: O(n)