# Selection Sort
Selection sort is a simple in-place comparison-based sorting algorithm.
In this method we divide the array in two sub-arrays :
- Sorted (left)
- Unsorted (right)
Initially we consider the whole array to be unsorted.
Select the leftmost element in the unsorted sub-array & swap it with the smallest element in the unsorted sub-array.
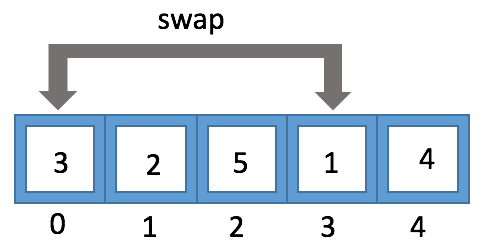
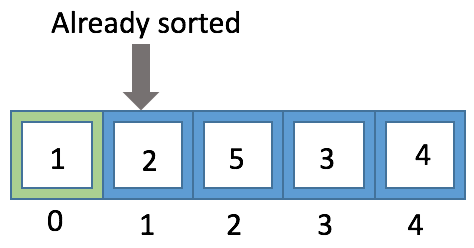
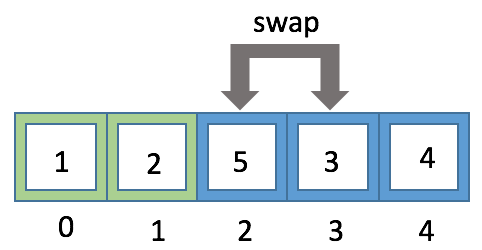
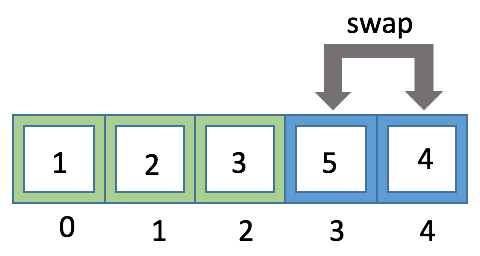
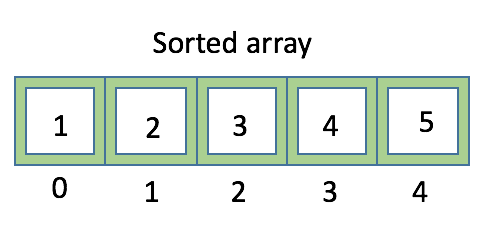
# Source Code - C++
#include <iostream>
using namespace std;
//Selection Sort Function
int selectionSort ( int A[], int n)
{
int i , j, small, temp;
for( i=0 ; i < n-1 ; i++)
{
small = i;
//finding smallest element in unsorted array
for( j=i+1 ; j < n ; j++)
{
if ( A[j] < A[small] )
small = j;
}
//swapping with left most unsorted element
temp = A[i];
A[i] = A[small];
A[small] = temp;
}
}
//Function to display Array elements
void displayArray(int A[],int n)
{
int i;
for(i=0;i<n;i++)
cout<<A[i]<<" "; cout<<"\n";
}
//Main Function
int main() {
int n;
cout<<"enter size of array\n"; cin>>n;
int A[n],e,i,ans;
cout<<"enter elements of array\n";
for(i=0;i<n;i++)
cin>>A[i]; cout<<"Array before sorting\n";
displayArray(A,n);
selectionSort(A,n);
cout<<"Array after sorting\n";
displayArray(A,n);
return 0;
}
Time Complexity O(n^2)