# Reverse a linked list
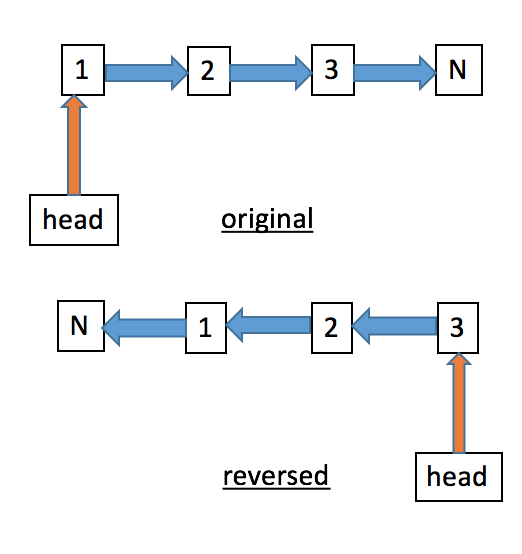
To reverse a linked list we will use three pointers:
p
- previous : To keep track of the previous Node.c
- current : To keet track of the current Node.n
- next : To keep track of the next Node.
Initialise
p
to NULL andc
equal to head that is it points to the first element.Make
n
equal to the next node of the current node by equating it to link part ofc
pointer.Remove the link between node pointed by
n
& node pointed byc
And create a backward link from the current node
c
top
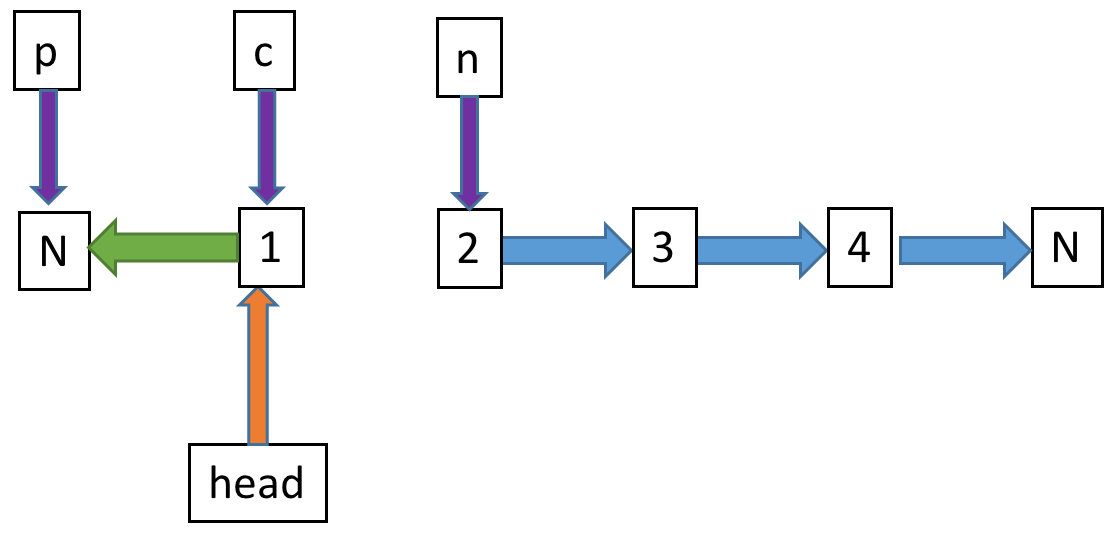
Make
p = c
& Movec
to the next node by equating it ton
.Repeat these steps for every node.
At the end equate head pointer to p to point it to the last node.
# Source Code - C++
#include <iostream>
using namespace std;
//Creating Node Structure
struct Node{
int data;
Node *link;
};
Node *head=NULL;
//Function to reverse linked list
void reverseList()
{
Node *p,*c,*n;
p=NULL;
c=head;
while(c!=NULL)
{
n=c->link;
c->link=p;
p=c;
c=n;
}
head=p;
}
//Function to insert at the end of linked list
void insertEnd (int d)
{
Node *ptr = new Node();
ptr->data=d;
ptr->link=NULL;
if(head==NULL)
head=ptr;
else
{
Node *temp = head;
while(temp->link != NULL)
{
temp=temp->link;
}
temp->link=ptr;
}
}
//Function to display linked list
void displayList()
{
Node *ptr=head;
while(ptr!=NULL)
{
cout<<ptr->data<<" ";
ptr=ptr->link;
}
cout<<"\n";
}
//Main Function
int main()
{
insertEnd(1);
insertEnd(2);
insertEnd(3);
insertEnd(4);
insertEnd(5);
displayList();
reverseList();
displayList();
return 0;
}
Time Complexity O(n)